In Angular, SPA stands for Single Page Application. It's a dynamic web app that loads a single HTML page and updates content without reloading the entire page. This provides a seamless experience, allowing you to navigate quickly through different views while maintaining application state. Angular enhances SPAs with features like two-way data binding, client-side routing, and efficient data handling via AJAX. Though SPAs offer a smoother experience, they may pose challenges, such as SEO and memory issues. Explore how Angular's unique capabilities can help you create effective SPAs and optimize user engagement.
Key Takeaways
- SPAs in Angular load a single HTML page and dynamically update content using JavaScript, enhancing user experience with smooth interactions.
- Angular's component-based architecture simplifies SPA development, enabling dynamic user interfaces with two-way data binding for real-time model-view synchronization.
- Client-side routing in Angular SPAs allows navigation without full page reloads, maintaining application state and improving performance efficiency.
- Angular provides built-in services for efficient API calls, streamlining data retrieval while reducing server load and bandwidth consumption.
- Implementing best practices like lazy loading and route guards in Angular SPAs enhances performance and security, while ensuring accessibility compliance.
Definition of Single Page Applications
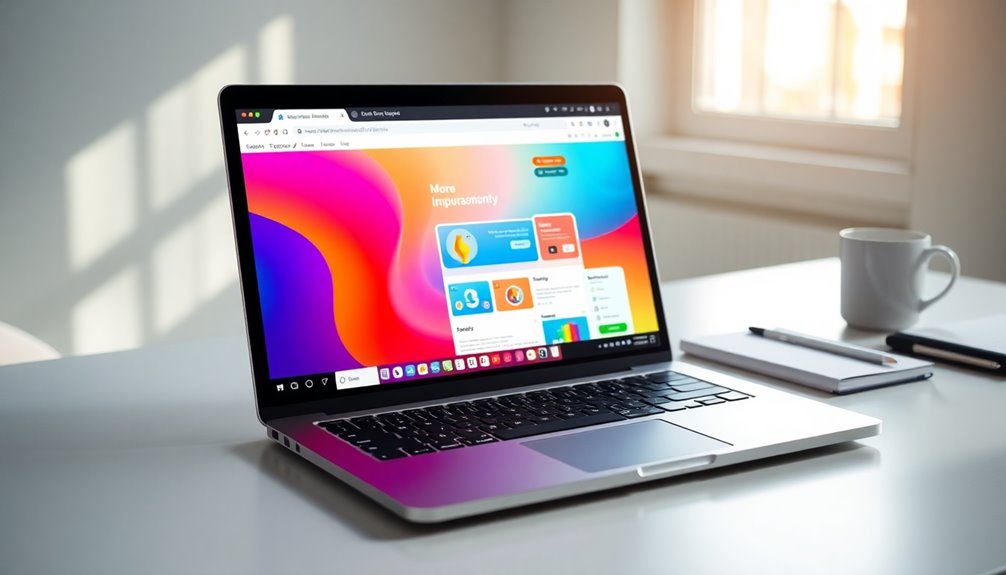
Single Page Applications (SPAs) are designed to load a single HTML page and dynamically update content, so you don't have to wait for full page reloads.
SPAs enhance the user experience by providing smoother interactions, primarily using JavaScript frameworks like Angular for client-side rendering. This allows efficient data fetching and rendering of views in response to your actions.
By relying on AJAX and HTML5 technologies, SPAs request and load new data asynchronously, minimizing server load and improving performance.
Additionally, SPAs manage URL changes through client-side routing, enabling you to navigate between views without reloading the entire application.
All applications built with Angular are classified as SPAs, thanks to their ability to render dynamic content while keeping the core HTML intact.
Key Features of SPAs
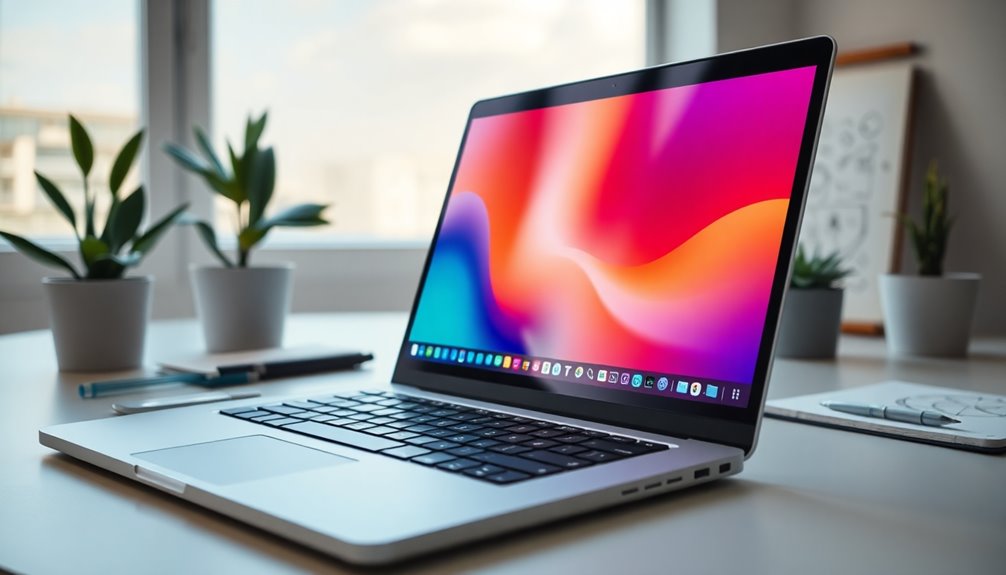
When you use a Single Page Application (SPA), you enjoy dynamic content loading that keeps your experience smooth and engaging.
Instead of waiting for entire pages to refresh, you get instant updates that make navigation feel seamless.
This enhanced user experience is one of the key reasons SPAs have become so popular. Additionally, the ability to optimize viewing conditions through techniques like lazy loading can significantly improve performance and responsiveness in SPAs.
Dynamic Content Loading
Dynamic content loading is a key feature that makes SPAs stand out, as it allows users to experience seamless interactions without the interruptions of full page reloads. By utilizing client-side routing, you can navigate between views while maintaining the application's core state. SPAs fetch data in lightweight formats like JSON, enabling rapid updates to the user interface. Angular enhances this process through two-way data binding, ensuring real-time updates between model and view. This approach minimizes server requests, loading only necessary resources upon interaction, thereby improving performance. Furthermore, the implementation of structured data can contribute to better search engine understanding of the content structure and relevance.
Feature | Benefit |
---|---|
Client-side Routing | Seamless navigation |
JSON Data Fetching | Faster updates |
Two-way Data Binding | Real-time synchronization |
Reduced Server Requests | Improved performance |
Enhanced Responsiveness | Better user experience |
Enhanced User Experience
Enhanced user experience is at the heart of what makes SPAs compelling, as they load a single HTML page and update content on the fly, allowing for faster interactions.
With Angular, you benefit from client-side routing, enabling seamless navigation between views while preserving application state. This means fewer server requests and a responsive design that keeps users engaged.
Two-way data binding enhances this experience by instantly reflecting changes in the user interface in the underlying data model.
Angular's component-based architecture fosters modularity and reusability, making your codebase cleaner and more maintainable.
Plus, lazy loading techniques guarantee that only necessary resources are loaded for the current view, further optimizing performance and improving user experience in your Single Page Applications.
Advantages of Using SPAs
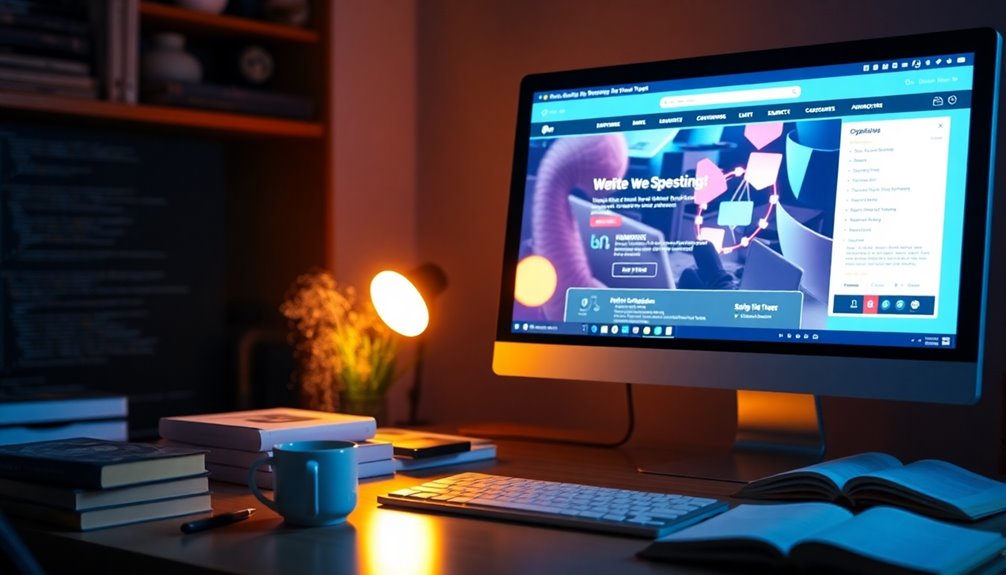
When you use a Single Page Application (SPA), you're stepping into a world of enhanced user experience and improved performance efficiency.
SPAs simplify the development process, allowing you to create applications that aren't only faster but also easier to maintain.
This combination makes SPAs a compelling choice for modern web development. Furthermore, the integration of AI-driven personal assistants is expected to streamline user interactions within SPAs, enhancing overall usability.
Enhanced User Experience
Single Page Applications (SPAs) greatly improve user experience by loading a single HTML page and dynamically updating content, which means you enjoy faster interactions and shorter wait times.
With Angular's component-based architecture, code reusability leads to quicker development cycles and consistent application behavior, enhancing your interactions even further.
SPAs utilize client-side routing to provide seamless shifts between different views, making navigation intuitive and engaging.
You won't face the interruptions typical of multi-page applications, creating a fluid and responsive web experience.
Additionally, reduced server load and lower bandwidth consumption mean the application runs more efficiently, allowing you to focus on what matters most—an enjoyable user experience with minimal delays.
Improved Performance Efficiency
By leveraging a single HTML page that updates dynamically, SPAs greatly boost performance efficiency.
Single Page Applications enhance your experience by minimizing full page reloads, resulting in faster shifts and improved performance.
With client-side routing, navigation occurs without constant server requests, creating a seamless user experience.
SPAs utilize AJAX and JSON for swift data retrieval, allowing for quick updates and rendering of dynamic content. This not only optimizes bandwidth usage but also helps reduce server load by fetching only necessary resources.
Furthermore, implementing lazy loading guarantees that components are loaded only when needed, resulting in faster initial load times and efficient use of system resources.
Simplified Development Process
The streamlined nature of Single Page Applications (SPAs) not only enhances performance but also simplifies the development process.
With Angular, you can create a single HTML page that dynamically updates content, minimizing the need for multiple files and creating a cleaner project structure.
Angular's component-based architecture allows you to build modular components that are easily maintained and reused throughout the application.
Thanks to two-way data binding, you can synchronize your model and view effortlessly, reducing boilerplate code and boosting productivity.
Client-side routing manages navigation without full page reloads, enhancing user experience and decreasing server load.
Plus, Angular's built-in services for API calls simplify data retrieval, letting you concentrate on application logic over repetitive code.
Disadvantages and Challenges
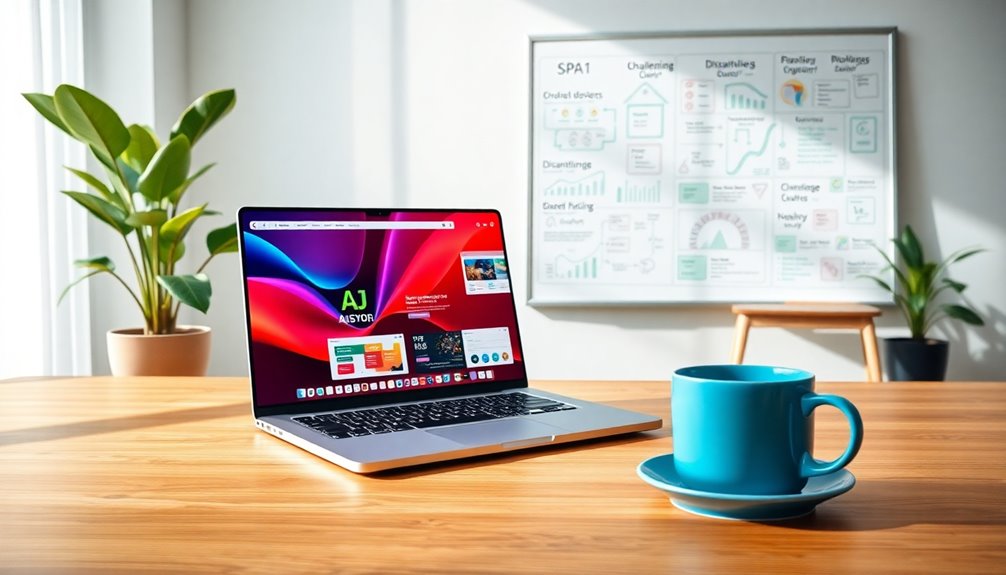
While SPAs in Angular offer a smooth user experience, they come with a set of distinct disadvantages and challenges that developers must navigate.
One major hurdle is SEO optimization; since SPAs rely heavily on JavaScript, search engines may struggle to index their static URLs effectively.
You might also face browser history management issues, leading to navigation problems for users who expect seamless shifts.
Additionally, security vulnerabilities like cross-site scripting necessitate rigorous input validation and sanitization of user-generated content.
High memory consumption can hinder performance, particularly on low-memory devices, as the entire application state stays in memory.
Finally, accessibility concerns emerge for users with disabled JavaScript, limiting their ability to access content and negatively impacting overall user experience.
Angular's Role in SPAs
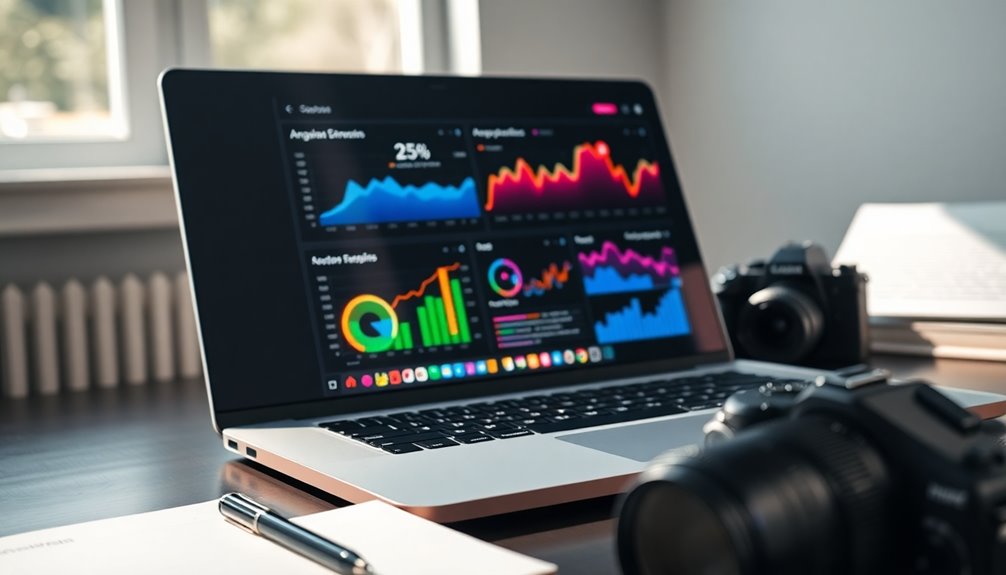
Angular plays an essential role in developing Single Page Applications (SPAs) by providing a robust framework that simplifies the process of creating dynamic, user-friendly interfaces.
As one of the leading Single Page Application Frameworks, it enables you to load dynamic content without loading new pages. Its component-based architecture promotes modularity, allowing you to build reusable components that encapsulate their own view and logic.
Angular's built-in routing capabilities facilitate seamless navigation between different views, enhancing the user experience. With two-way data binding, any changes in the model instantly reflect in the view, providing real-time updates.
Additionally, Angular's dependency injection system improves testability and state management, making it ideal for complex SPAs.
Creating an Angular SPA
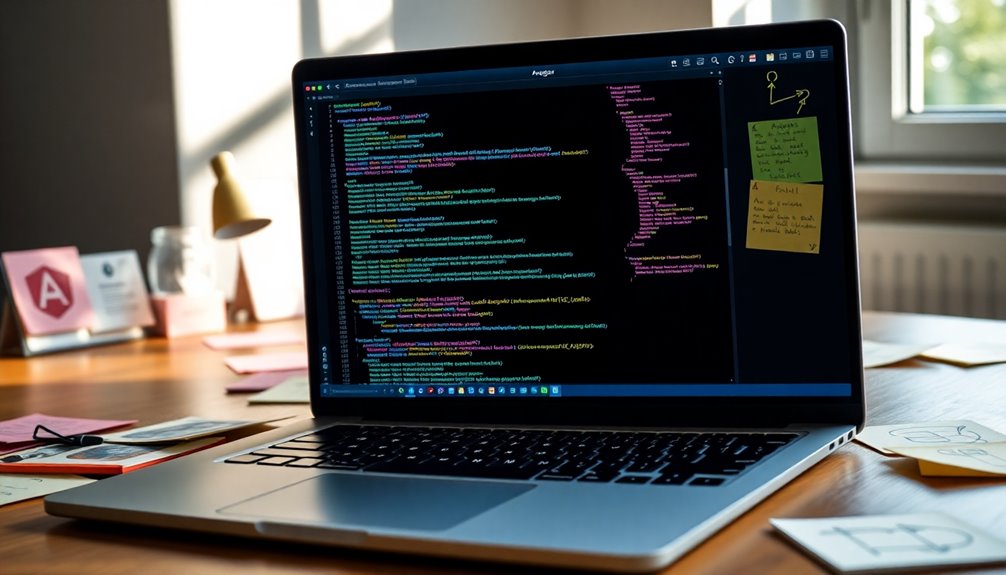
To create an Angular Single Page Application (SPA), you'll want to start by using the Angular CLI, which simplifies project setup and allows for easy initialization of routing.
After generating your project, define application routes in a dedicated routing module, specifying paths and associated components. This setup enables navigation without full page reloads.
Next, implement the '
Utilize the RouterLink directive to create navigation links, enhancing user experience as users shift between views.
Finally, include a wildcard route, like '{path: '', component: PageNotFoundComponent**}', to handle undefined routes and provide feedback for non-existent pages.
Routing in Angular Applications
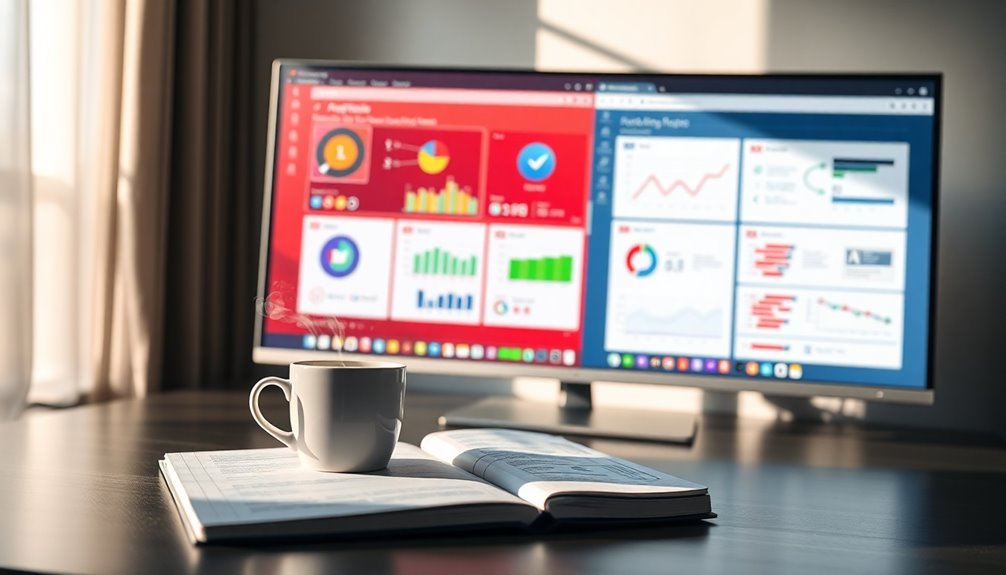
When building a Single Page Application (SPA), effective routing is essential for creating a seamless user experience. Angular's routing, managed through the '@angular/router' module, allows you to navigate between different components without full page reloads.
You define routes in a dedicated routing module, specifying each 'path' and its corresponding 'component'. The 'router-outlet' directive in your main application template dynamically renders the active component based on the current route.
To enhance application performance, Angular supports lazy loading, ensuring components load only when needed during user navigation. Additionally, you can implement route guards to restrict access to certain routes based on authentication, enhancing both security and user experience within your application.
Best Practices for SPAs
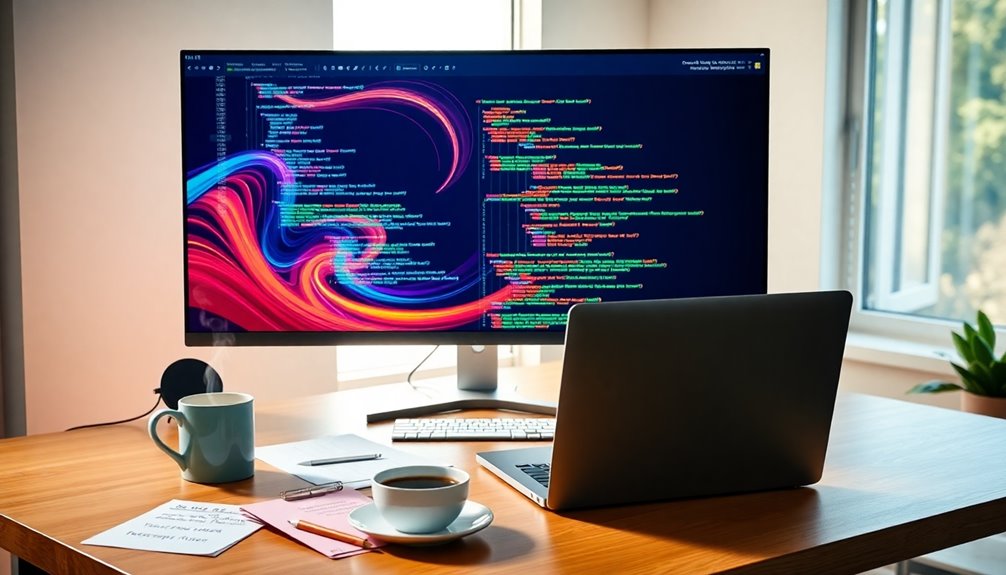
Building a successful Single Page Application (SPA) requires adhering to best practices that enhance performance, security, and user experience.
Start by optimizing application performance with lazy loading, which only loads necessary modules when required, improving initial load times. Implement route guards to protect sensitive data by controlling access based on user authentication.
Efficient state management using libraries like NgRx or Akita guarantees a predictable data flow.
Don't overlook SEO strategies; utilize Angular Universal for server-side rendering to help search engines index your dynamic content effectively.
Finally, verify accessibility compliance by following best practices like using ARIA roles and maintaining keyboard navigability, making your SPA usable for everyone.
These practices will greatly boost your application's effectiveness and reach.
Frequently Asked Questions
What Is Single Page Application SPAS?
Imagine a world where every click takes you on a delightful journey without the annoying wait for new pages to load.
That's what single page applications (SPAs) offer. Instead of reloading the entire site, they dynamically update content, making your experience seamless and fast.
You get instant access to information without the frustrating delays. SPAs use technologies to keep things smooth, ensuring you stay engaged and entertained while browsing your favorite sites.
What Is SPA and How Does It Work?
A Single Page Application (SPA) is a web application that loads a single HTML page and dynamically updates content without full page reloads.
You interact with it seamlessly, as it uses JavaScript to fetch data and render views. When you navigate, the URL updates, but the page doesn't refresh.
This approach improves performance and user experience, making your interactions feel faster and more fluid while maintaining a consistent look and feel throughout the application.
What Is the SPA Concept?
Imagine a world where every click feels like magic—no waiting, no loading screens, just instant content!
That's the essence of the SPA concept. You're diving into a single-page application that loads once and updates dynamically as you navigate.
Instead of reloading the whole page, it fetches only the data you need, keeping your experience smooth and seamless.
It's like flipping through a book without turning the pages—effortless and engaging!
What Is Difference Between SPA and Web Application?
The main difference between a Single Page Application (SPA) and a traditional web application lies in how they handle content updates.
In a SPA, you interact with a single HTML page that dynamically changes without reloading, providing a smoother experience.
In contrast, traditional web applications refresh the entire page for each action, which can slow you down.
SPAs often use AJAX for seamless data fetching, enhancing user engagement and reducing frustration.
Conclusion
In the world of web development, SPAs are like rivers, flowing seamlessly from one experience to another. As you embrace Angular, you're crafting a vibrant landscape where users can navigate effortlessly. With the right tools and best practices, you're not just building applications; you're creating a journey. So, plunge into and let your creativity shape the currents of your SPA, ensuring every interaction is smooth and engaging, just like a gentle breeze guiding a sailboat across the water.